Implementing a CI/CD Pipeline for Kubernetes Applications with Jenkins and Helm
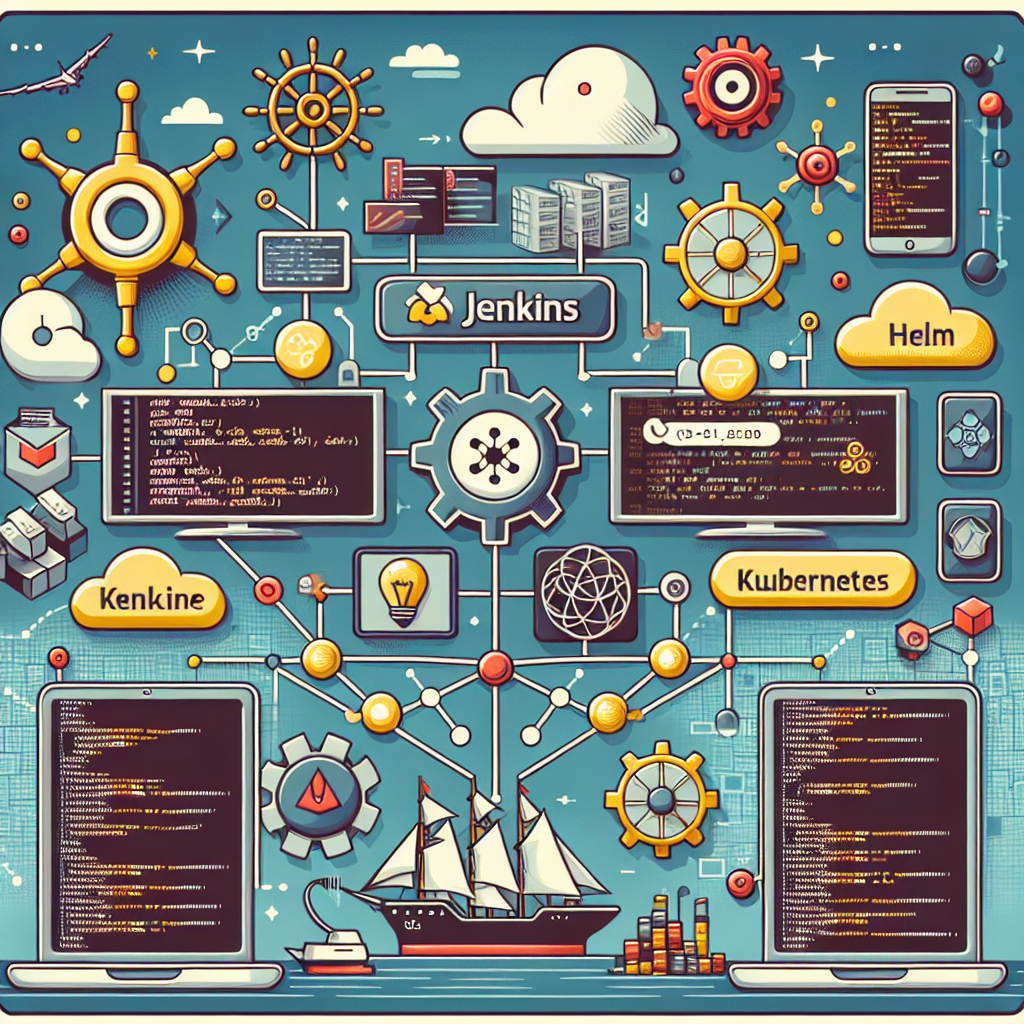
With the exponential rise in cloud-native architectures, orchestrating and managing applications in cloud environments has become more sophisticated than ever. One of the core tools that has empowered this transition is Kubernetes. Kubernetes automates deployment, scaling, and operations of application containers across clusters of hosts, providing container-centric infrastructure. In this post, we will dive into the subject of setting up a CI/CD pipeline for a Kubernetes application using Jenkins and Helm.
Why CI/CD with Kubernetes?
Continuous Integration and Continuous Deployment (CI/CD) pipelines are essential for accelerating software delivery while ensuring high quality and stability of applications. When integrated with Kubernetes, a CI/CD pipeline can streamline the development lifecycle, allowing for seamless deployment and robust management of application versions.
Prerequisites
Before we get started, ensure you have the following prerequisites:
- A Kubernetes cluster (local or cloud-based)
- kubectl CLI tool installed
- Helm installed
- A Jenkins server
Step-by-Step Guide
1. Setting Up Your Kubernetes Cluster
If you don't have a Kubernetes cluster yet, you can set one up locally using minikube:
minikube start
For cloud-based options, you could use services like EKS (AWS), GKE (Google Cloud), or AKS (Azure).
2. Installing Helm
Helm helps you manage Kubernetes applications. To install Helm, run the following script:
curl https://raw.githubusercontent.com/helm/helm/master/scripts/get-helm-3 | bash
3. Setting Up Jenkins
Install Jenkins by following the official documentation. Once installed, set up a simple job to ensure it can interact with your Kubernetes cluster. Install necessary plugins like Kubernetes Continuous Deploy and Pipeline: AWS Steps.
4. Preparing Your Helm Chart
Create a Helm chart for your application. For example, generate a new Helm chart using:
helm create my-app
Customize the generated files to match your application's configurations.
5. Writing Jenkins Pipeline Script
Create a Jenkinsfile in your repository's root directory. This file defines your CI/CD pipeline. Here's a simple example:
pipeline {
agent any
environment {
KUBECONFIG_CREDENTIALS_ID = 'kubeconfig-id'
}
stages {
stage('Checkout') {
steps {
git 'https://github.com/your-repo/your-app.git'
}
}
stage('Build') {
steps {
sh 'echo "Building the application..."'
sh 'mvn clean package' // Replace with your build commands
}
}
stage('Docker Build') {
steps {
script {
dockerImage = docker.build("your-docker-repo/your-app:${env.BUILD_ID}")
}
}
}
stage('Push Image') {
steps {
script {
dockerImage.push()
dockerImage.push('latest')
}
}
}
stage('Deploy') {
steps {
withKubeConfig([credentialsId: env.KUBECONFIG_CREDENTIALS_ID]) {
sh 'helm upgrade --install my-app ./helm/my-app'
}
}
}
}
post {
always {
cleanWs()
}
}
}
6. Configuring Kubeconfig in Jenkins
Ensure that Jenkins has access to your Kubernetes cluster by configuring kubeconfig. You can add your kubeconfig
file under Manage Jenkins > Configure System > Kubernetes CLI Plugin.
7. Running the Pipeline
Push your changes to your repository and start the pipeline. If configured correctly, Jenkins will:
- Checkout the repository
- Build the application
- Build and push Docker image
- Deploy the application to the Kubernetes cluster using Helm
Best Practices and Lessons Learned
1. Secure Your Secrets
Use Kubernetes secrets and Jenkins credentials to secure sensitive data like API keys and tokens. Avoid hardcoding secrets in your scripts.
2. Modularize Your Helm Charts
Organize your Helm charts with a clear structure, separating configuration values from templates. Leverage reusable sub-charts whenever possible.
3. Monitor Deployments
Implement monitoring and alerting for your deployments using tools like Prometheus and Grafana. This ensures you can respond quickly to any issues that arise post-deployment.
4. Rollback Strategy
Configure Helm to enable rolling back to previous stable versions in case of a failed deployment. This minimizes downtime and ensures quick recovery.
Conclusion
Setting up a CI/CD pipeline with Jenkins and Helm for Kubernetes applications can significantly enhance your software delivery process. This integration not only accelerates the release cycle but also ensures robust and reliable deployments. Have you implemented a CI/CD pipeline for your Kubernetes applications? Share your successes and challenges in the comments below!