Getting Started with Terraform: Automating AWS Infrastructure Provisioning
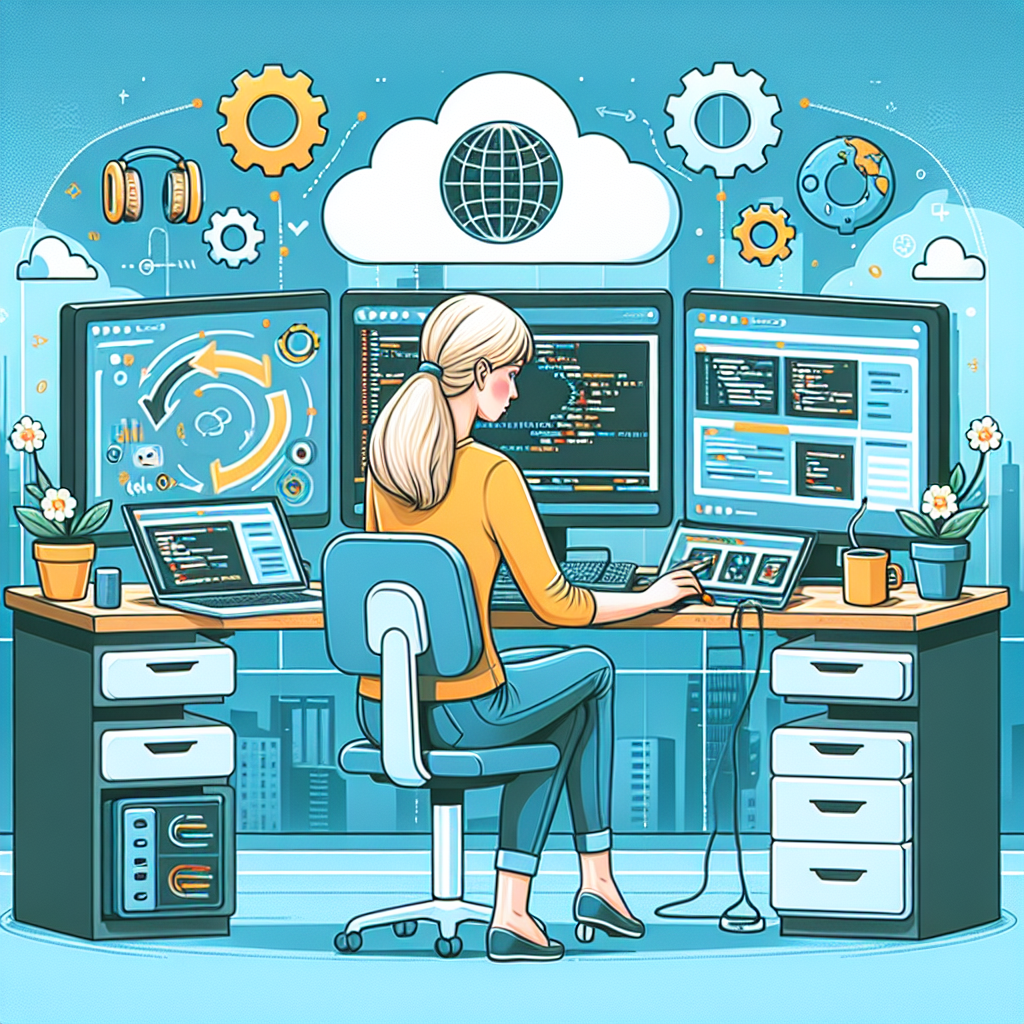
With the increasing adoption of cloud-native technologies, infrastructure as code (IaC) has become a key practice for deploying and managing cloud resources. One of the most powerful tools in the IaC landscape is Terraform. In this blog post, we'll explore how to use Terraform to provision cloud infrastructure on AWS, enabling you to automate and efficiently manage your cloud resources. We'll include practical examples to help you get started.
Why Terraform?
Terraform, developed by HashiCorp, is an open-source IaC tool that allows you to define and provision infrastructure in a highly consistent and reusable fashion. Key benefits of using Terraform include:
- Provider Agnostic: Supports multiple cloud providers, including AWS, Azure, GCP, and more, allowing the same codebase to manage different environments.
- Declarative Configuration: Infrastructure is defined in a high-level configuration language, making it easy to understand and maintain.
- Version Control: Infrastructure definitions can be stored in version control systems, enabling collaboration and change tracking.
- State Management: Terraform maintains the state of your infrastructure, ensuring that the desired and current states are synchronized.
- Dependency Management: Automatically manages resource dependencies, ensuring that resources are provisioned in the correct order.
Getting Started with Terraform on AWS
Let's walk through the steps to set up Terraform and use it to provision a simple infrastructure on AWS.
Step 1: Install Terraform
First, you need to install Terraform on your local machine. You can download the appropriate package from the Terraform website and follow the installation instructions for your operating system.
Step 2: Configure AWS Credentials
Terraform requires AWS credentials to provision resources. If you haven't already, install the AWS Command Line Interface (CLI) and configure your credentials:
aws configure
You'll be prompted to enter your AWS Access Key, Secret Access Key, region, and output format.
Step 3: Define Your Infrastructure
Create a new directory for your Terraform configuration files. Inside this directory, create a file named main.tf
and define your infrastructure. For this example, let's create a simple EC2 instance:
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "ExampleInstance"
}
}
Step 4: Initialize Terraform
Navigate to your configuration directory and run the following command to initialize Terraform. This command prepares your working directory by downloading the necessary provider plugins.
terraform init
Step 5: Plan Your Infrastructure
Before applying your configuration, you can run the terraform plan
command to see what actions Terraform will perform. This helps in understanding the changes before applying them:
terraform plan
Step 6: Apply Your Configuration
Now, apply your configuration to provision the resources defined in main.tf
:
terraform apply
You'll be prompted to confirm the action. Type "yes" to proceed. Terraform will create the EC2 instance as specified.
Step 7: Inspect Your Infrastructure
Once the apply process completes, you can verify the resources in the AWS Management Console. You should see the new EC2 instance under the specified region with the given name.
Best Practices for Using Terraform
Here are some best practices to follow when using Terraform to manage your infrastructure:
1. Organize Your Configuration Files
As your infrastructure grows, organize your configuration files into modules and directories. This improves maintainability and reusability. For example:
├── main.tf
├── variables.tf
├── outputs.tf
└── modules/
├── vpc/
├── ec2/
└── rds/
2. Use Variables and Outputs
Define variables and outputs in separate files (variables.tf
and outputs.tf
) to make your configurations more flexible and easier to manage:
# variables.tf
variable "instance_type" {
description = "Type of instance to create"
default = "t2.micro"
}
# outputs.tf
output "instance_id" {
description = "The ID of the EC2 instance"
value = aws_instance.example.id
}
3. Use Remote State Storage
Store Terraform state files remotely using backends like Amazon S3, which supports state locking and consistency checks:
terraform {
backend "s3" {
bucket = "my-terraform-state"
key = "state/terraform.tfstate"
region = "us-east-1"
}
}
4. Implement Infrastructure Testing
Use tools like Terratest to write automated tests for your infrastructure, ensuring configurations work as expected and reducing the risk of misconfigurations.
Conclusion
Terraform is a powerful tool for managing infrastructure as code, providing a consistent and efficient way to provision and maintain cloud resources. By following the steps and best practices outlined in this post, you can start using Terraform to automate your AWS infrastructure, leading to more predictable and scalable deployments. Have you used Terraform in your cloud-native projects? Share your experiences and tips in the comments below!