Getting Started with AWS Lambda: Building and Deploying Your First Serverless Function
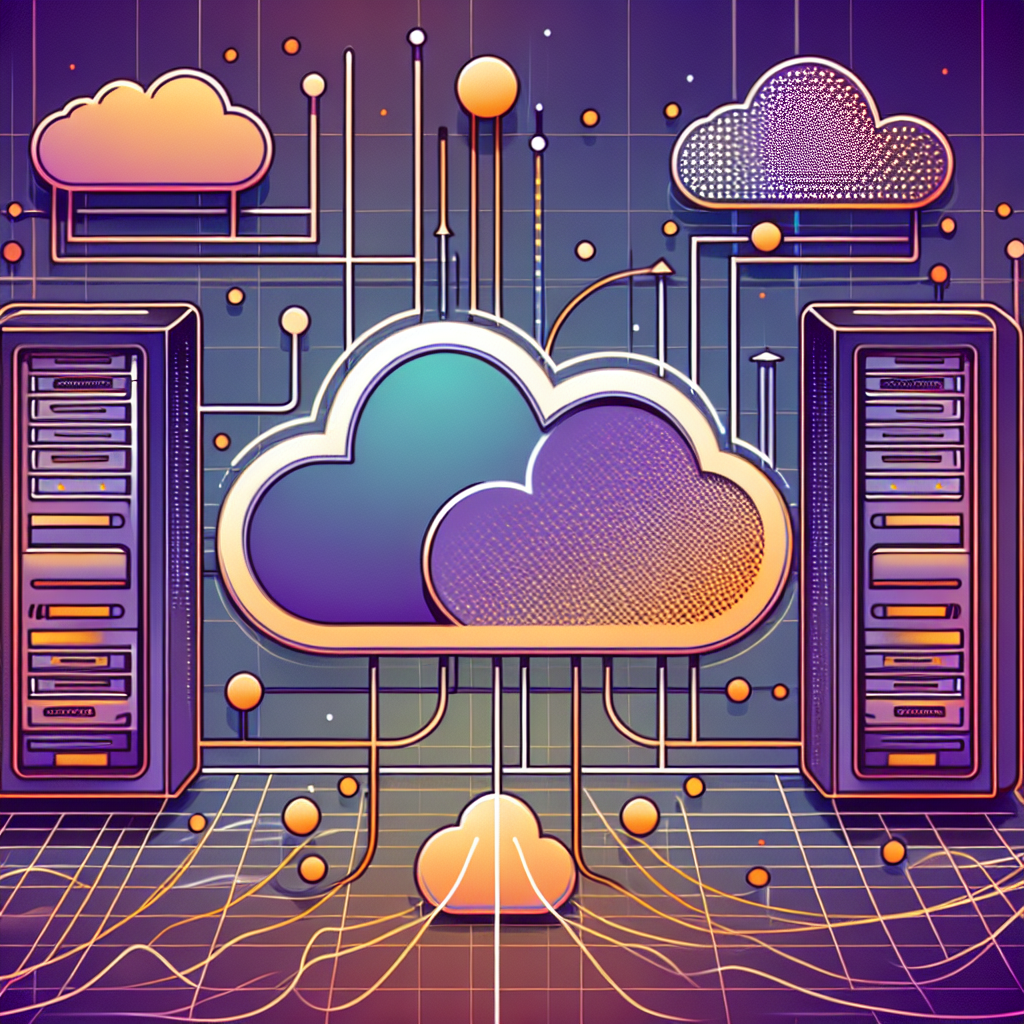
Serverless computing is revolutionizing the way developers build and deploy applications. By abstracting the underlying infrastructure, serverless platforms allow you to focus solely on writing code. One of the most popular serverless platforms is AWS Lambda, which enables you to run code in response to events without provisioning or managing servers. In this blog post, we'll dive into AWS Lambda, exploring how to create, deploy, and monitor a serverless function using the AWS CLI and the AWS Management Console.
Introduction to AWS Lambda
AWS Lambda is a compute service that lets you run code without provisioning or managing servers. It executes your code only when needed and scales automatically, from a few requests per day to thousands per second. You pay only for the compute time you consume—there’s no charge when your code isn’t running.
Creating a Lambda Function
Let's start by creating a simple Lambda function that processes events from an Amazon S3 bucket. This function will be triggered whenever a new object is uploaded to the specified bucket.
1. Set Up Your Development Environment
Ensure you have the AWS CLI installed and configured with the appropriate permissions to create and manage Lambda functions.
aws configure
Create a new directory for your Lambda function:
mkdir lambda-s3-trigger
cd lambda-s3-trigger
2. Write the Lambda Function Code
Create a file named lambda_function.py
with the following content:
import json
def lambda_handler(event, context):
for record in event['Records']:
s3 = record['s3']
bucket = s3['bucket']['name']
key = s3['object']['key']
print(f"New object added to bucket {bucket}: {key}")
return {
'statusCode': 200,
'body': json.dumps('Successfully processed S3 event')
}
3. Create a Deployment Package
Package your Lambda function code into a zip file:
zip function.zip lambda_function.py
4. Create the Lambda Function Using AWS CLI
Run the following command to create the Lambda function, replacing YOUR_ROLE_ARN
with your AWS IAM role ARN:
aws lambda create-function \
--function-name ProcessS3Event \
--zip-file fileb://function.zip \
--handler lambda_function.lambda_handler \
--runtime python3.8 \
--role YOUR_ROLE_ARN
5. Configure S3 Event Trigger
Now, set up an S3 bucket event notification to trigger your Lambda function whenever a new object is uploaded. Replace YOUR_BUCKET_NAME
with your S3 bucket name:
aws s3api put-bucket-notification-configuration \
--bucket YOUR_BUCKET_NAME \
--notification-configuration '{
"LambdaFunctionConfigurations": [
{
"LambdaFunctionArn": "arn:aws:lambda:us-east-1:123456789012:function:ProcessS3Event",
"Events": ["s3:ObjectCreated:*"]
}
]
}'
Ensure you grant the S3 bucket permission to invoke your Lambda function:
aws lambda add-permission \
--function-name ProcessS3Event \
--principal s3.amazonaws.com \
--statement-id s3invoke \
--action "lambda:InvokeFunction" \
--source-arn arn:aws:s3:::YOUR_BUCKET_NAME \
--source-account YOUR_ACCOUNT_ID
Testing the Lambda Function
To test your Lambda function, you can upload a file to the S3 bucket. This should trigger the Lambda function and output a log message indicating that a new object has been processed.
aws s3 cp test-file.txt s3://YOUR_BUCKET_NAME/
Check the logs of your Lambda function using the AWS Management Console or the AWS CLI:
aws logs tail /aws/lambda/ProcessS3Event --follow
You should see a log entry similar to:
New object added to bucket YOUR_BUCKET_NAME: test-file.txt
Monitoring and Troubleshooting
Monitoring is crucial to ensure your Lambda functions perform as expected. AWS provides several tools for monitoring and troubleshooting Lambda functions:
- Amazon CloudWatch: View logs, set alarms, and monitor your Lambda function's performance.
- AWS X-Ray: Visualize the end-to-end tracing of your requests and identify performance bottlenecks.
Use the following command to enable X-Ray tracing for your Lambda function:
aws lambda update-function-configuration \
--function-name ProcessS3Event \
--tracing-config Mode=Active
Common Pitfalls and Best Practices
While AWS Lambda offers numerous benefits, there are common pitfalls to be aware of:
- Cold Start Latency: Minimize the impact of cold starts by ensuring your handler code is optimized and dependencies are initialized efficiently.
- Resource Limits: Be mindful of AWS Lambda's memory and execution time limits. Optimize your code to stay within these limits.
- Error Handling: Implement comprehensive error handling and retries for robust and resilient functions.
Conclusion
Serverless computing with AWS Lambda allows you to build scalable and efficient applications without the burden of managing infrastructure. By following the steps outlined in this post, you can create, deploy, and monitor Lambda functions to handle various event-driven use cases. Embrace serverless architecture to streamline your development process and focus on what matters most—delivering value to your users. Have you built successful serverless applications using AWS Lambda? Share your experiences and tips in the comments below!