Extending Kubernetes with Custom Kubectl Plugins: Automate and Simplify Your Workflows
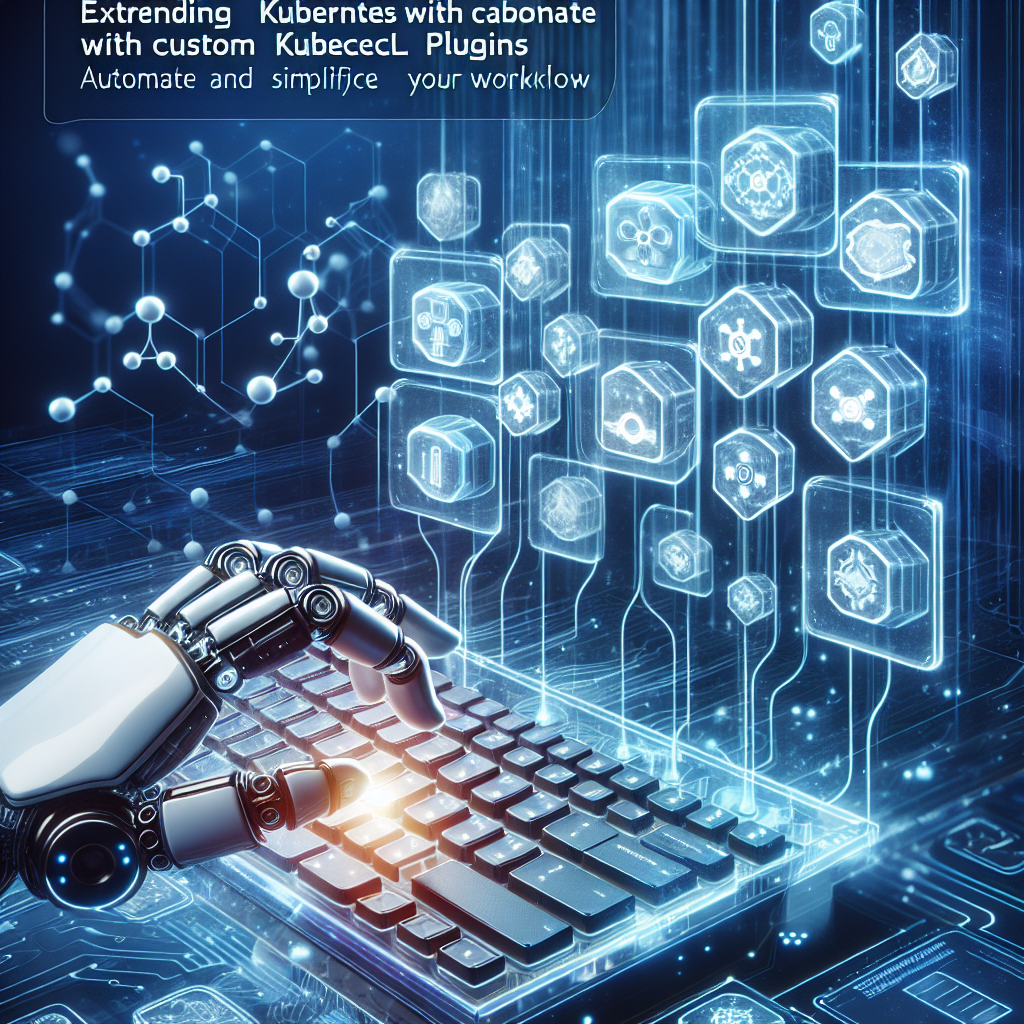
In the realm of cloud-native technologies, Kubernetes has emerged as the de facto standard for container orchestration. It simplifies the deployment, scaling, and management of containerized applications. However, managing Kubernetes clusters and resources can become complex, especially when you need to automate repetitive tasks or integrate with CI/CD pipelines. That’s where kubectl plugins come into play. In this blog post, we’ll explore how to create custom kubectl plugins to extend Kubernetes functionality and automate workflows.
What Are Kubectl Plugins?
Kubectl plugins are external programs that are invoked by the kubectl command. These plugins allow you to add custom commands to kubectl, making it easier to automate tasks or encapsulate complex operations in reusable commands. Kubectl plugins can be written in any programming language that can interact with Kubernetes API.
Why Use Kubectl Plugins?
Using kubectl plugins offers several benefits:
- Automation: Simplify and automate repetitive tasks.
- Customization: Extend kubectl to meet your specific needs.
- Reusability: Encapsulate complex operations in reusable commands.
- Integration: Integrate with other tools and workflows.
Creating Your First Kubectl Plugin
We’ll walk through the steps to create a simple kubectl plugin that retrieves and displays the number of pods running in all namespaces.
1. Set Up Your Development Environment
First, ensure that you have kubectl installed and configured on your system. You will also need a programming language to write your plugin; in this example, we’ll use Python.
2. Write the Plugin Script
Create a file named kubectl-podcount
with the following Python script:
#!/usr/bin/env python
import subprocess
import json
def get_pod_count():
result = subprocess.run(
["kubectl", "get", "pods", "--all-namespaces", "-o", "json"],
stdout=subprocess.PIPE,
check=True
)
pods = json.loads(result.stdout)
return len(pods['items'])
if __name__ == "__main__":
pod_count = get_pod_count()
print(f"Total number of pods running: {pod_count}")
3. Make the Script Executable
Make the script executable by running:
chmod +x kubectl-podcount
4. Add the Script to Your PATH
Move the script to a directory that is in your PATH, for example:
mv kubectl-podcount /usr/local/bin/
5. Test the Plugin
You can now run your custom kubectl plugin by executing:
kubectl podcount
You should see the total number of pods running in all namespaces.
Advanced Kubectl Plugin Example: Automatically Labeling Namespaces
Let’s create a more advanced plugin that automatically labels all namespaces with a custom label. This is useful for scenarios where you need to ensure consistency across multiple environments.
1. Write the Plugin Script
Create a file named kubectl-label-namespaces
with the following Python script:
#!/usr/bin/env python
import subprocess
import json
import sys
def label_namespaces(label_key, label_value):
result = subprocess.run(
["kubectl", "get", "namespaces", "-o", "json"],
stdout=subprocess.PIPE,
check=True
)
namespaces = json.loads(result.stdout)
for ns in namespaces['items']:
ns_name = ns['metadata']['name']
label = f"{label_key}={label_value}"
subprocess.run(
["kubectl", "label", "namespace", ns_name, label, "--overwrite"],
check=True
)
print(f"Labeled namespace {ns_name} with {label}")
if __name__ == "__main__":
if len(sys.argv) != 3:
print("Usage: kubectl label-namespaces ")
sys.exit(1)
label_key = sys.argv[1]
label_value = sys.argv[2]
label_namespaces(label_key, label_value)
2. Make the Script Executable
Make the script executable by running:
chmod +x kubectl-label-namespaces
3. Add the Script to Your PATH
Move the script to a directory that is in your PATH, for example:
mv kubectl-label-namespaces /usr/local/bin/
4. Test the Plugin
You can now run your custom kubectl plugin by executing:
kubectl label-namespaces environment production
You should see output indicating that each namespace has been labeled with environment=production
.
Conclusion
Kubectl plugins are a powerful way to extend the functionality of Kubernetes and automate tasks. By creating custom plugins, you can tailor your Kubernetes experience to fit your workflow and requirements. Start experimenting with kubectl plugins today and share your unique creations in the comments below!