Extend Your Kubernetes Toolbox: Creating Custom Kubectl Plugins
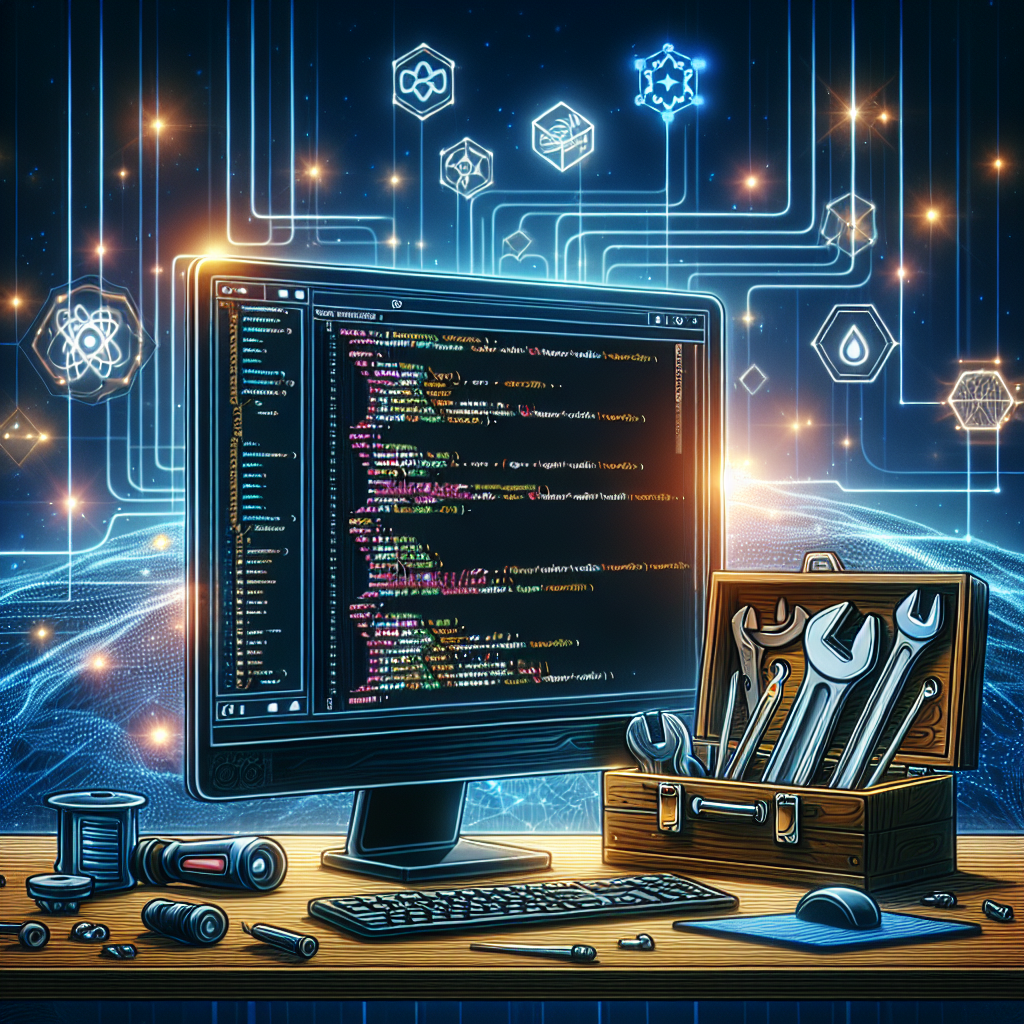
As cloud-native technologies continue to evolve, Kubernetes has become the go-to platform for container orchestration. One of the key aspects of managing Kubernetes clusters efficiently is to simplify interactions and automate repetitive tasks. In this post, we’ll explore how to use Kubectl Plugins to extend and customize Kubernetes command-line functionality.
What Are Kubectl Plugins?
Kubectl is the command-line tool for interacting with Kubernetes clusters. While it comes with a set of built-in commands, it has a plugin system that allows you to create custom commands to extend its functionality. These plugins can be written in any programming language that can execute from a command line, making them highly versatile.
Setting Up Your Environment
Before you start writing your first kubectl plugin, make sure you have the following installed:
Creating Your First Kubectl Plugin
Let’s create a simple kubectl plugin to list all pods with their namespaces. Follow these steps:
1. Create the Plugin Script
Create a directory for your plugin, let's call it kubectl-plugins
. Inside this directory, create a shell script named kubectl-pods-ns
:
mkdir -p ~/.kubectl/plugins
cd ~/.kubectl/plugins
touch kubectl-pods-ns
chmod +x kubectl-pods-ns
Edit the kubectl-pods-ns
file with the following content:
#!/bin/bash
kubectl get pods --all-namespaces -o=custom-columns=NAME:.metadata.name,NAMESPACE:.metadata.namespace
2. Make the Plugin Executable
Ensure that your script is executable:
chmod +x kubectl-pods-ns
3. Adding the Plugin to Your PATH
To make the plugin accessible anywhere in your terminal, add its directory to your PATH
environment variable. Add the following line to your .bashrc
, .zshrc
, or appropriate terminal configuration file:
export PATH=$PATH:~/.kubectl/plugins
After adding the line, apply the changes:
source ~/.bashrc # or source ~/.zshrc
4. Running Your Plugin
You can now run your kubectl plugin with the following command:
kubectl pods-ns
This will list all pods across all namespaces in your Kubernetes cluster.
Advanced Plugins with Python
For more complex plugins, you might want to use a scripting language like Python. Let’s create a kubectl plugin that provides detailed node resource usage statistics using kubectl top
:
1. Create the Python Script
Create a script named kubectl-node-stats
in the same ~/.kubectl/plugins
directory:
touch kubectl-node-stats
chmod +x kubectl-node-stats
Edit the kubectl-node-stats
file with the following Python code:
#!/usr/bin/env python3
import subprocess
import json
def get_node_stats():
command = ['kubectl', 'top', 'nodes', '--no-headers']
result = subprocess.run(command, stdout=subprocess.PIPE, check=True)
output = result.stdout.decode('utf-8').splitlines()
nodes = []
for line in output:
columns = line.split()
node_stats = {
'name': columns[0],
'cpu': columns[1],
'memory': columns[3]
}
nodes.append(node_stats)
return nodes
def main():
nodes = get_node_stats()
print(json.dumps(nodes, indent=2))
if __name__ == "__main__":
main()
2. Make the Script Executable
Ensure the Python script is executable:
chmod +x kubectl-node-stats
3. Running the Advanced Plugin
You can now run this advanced plugin with the command:
kubectl node-stats
This plugin will output node resource usage statistics in JSON format, making it easily parsable and useful for further automation.
Lessons Learned and Best Practices
While creating and using kubectl plugins, keep the following in mind:
- Modularity: Keep your plugins modular and focused on single tasks.
- Error Handling: Implement robust error handling to make your plugins reliable.
- Documentation: Document your plugins to help your team understand and use them effectively.
- Version Control: Use version control (e.g., Git) to manage and track changes to your plugins.
Conclusion
Kubectl plugins are a powerful way to enhance your Kubernetes command-line experience by creating custom commands tailored to your workflow. Whether you're writing simple scripts or advanced programs, the ability to extend kubectl can significantly boost your productivity. Start creating your own plugins today and share your experiences in the comments below!