Building CI/CD Pipelines with Jenkins and Kubernetes: A Comprehensive Guide
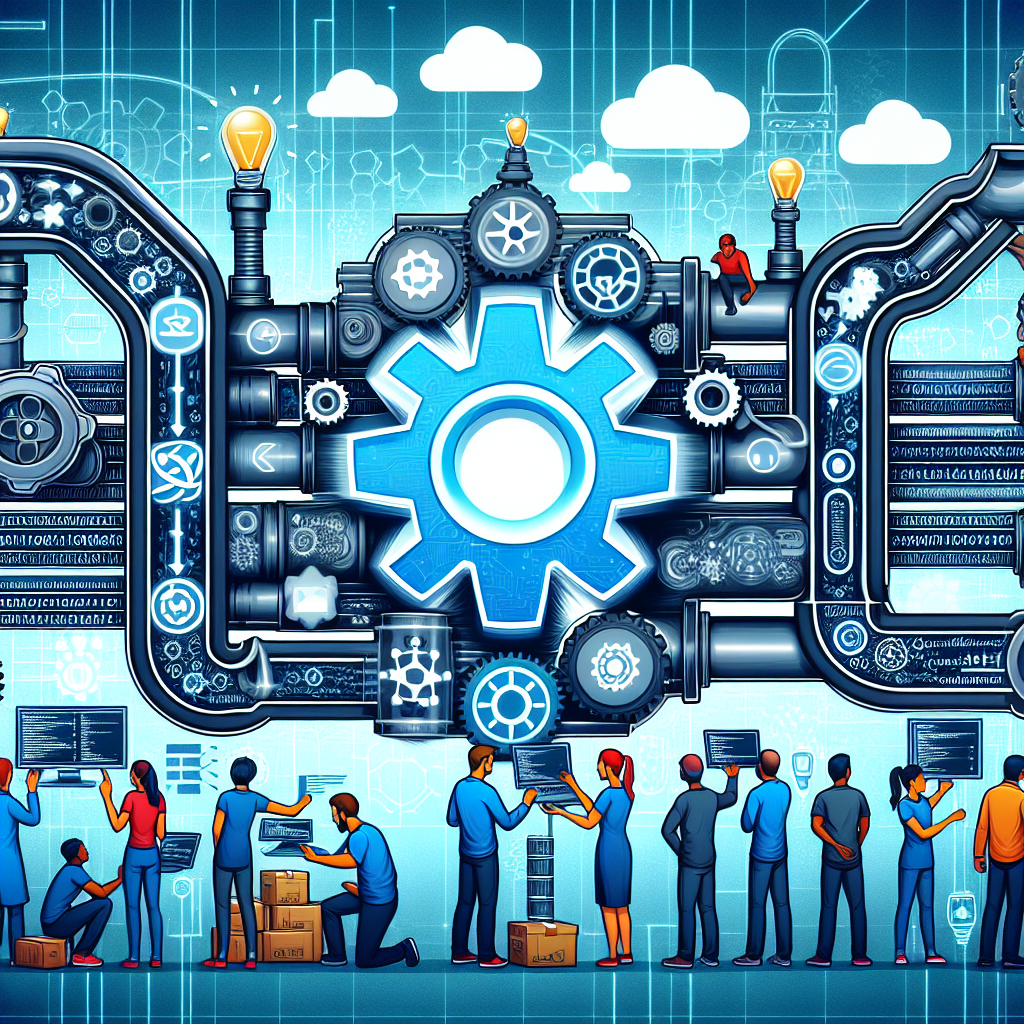
In today's fast-paced software development landscape, continuous integration and continuous deployment (CI/CD) pipelines are crucial for efficient and reliable software delivery. Tools like Jenkins play a pivotal role in automating the CI/CD process. When combined with Kubernetes, Jenkins can drive powerful and scalable automated pipelines. In this blog post, we'll explore how to set up Jenkins in a Kubernetes cluster using Helm and create a CI/CD pipeline for a sample application. We'll also share best practices and lessons learned.
Setting Up Jenkins in Kubernetes
To get started, we need to deploy Jenkins in our Kubernetes cluster. We'll use Helm, a package manager for Kubernetes, to simplify this process.
1. Prerequisites
- An existing Kubernetes cluster
- The Kubernetes command-line tool (kubectl) configured to communicate with your cluster
- Helm installed
2. Install Jenkins using Helm
First, add the Jenkins Helm chart repository:
helm repo add jenkins https://charts.jenkins.io
helm repo update
Next, create a namespace for Jenkins and install Jenkins using Helm:
kubectl create namespace jenkins
helm install jenkins jenkins/jenkins --namespace jenkins
This command installs Jenkins in the jenkins
namespace. To get the initial admin password, run:
kubectl get secret --namespace jenkins jenkins -o jsonpath="{.data.jenkins-admin-password}" | base64 --decode
Access Jenkins using a web browser. Run the following command to get the Jenkins URL:
kubectl --namespace jenkins get svc jenkins
Navigate to the Jenkins URL and log in using the admin username admin
and the password obtained from the previous command.
Creating a CI/CD Pipeline
Now that Jenkins is set up, let's create a CI/CD pipeline for a sample application. We'll use a simple Node.js application for demonstration purposes.
1. Create a New Pipeline
In Jenkins, click on "New Item," enter a name for your pipeline, select "Pipeline," and click "OK."
2. Configure the Pipeline
In the pipeline configuration, scroll down to the "Pipeline" section and select "Pipeline script from SCM." Configure the following:
- SCM: Git
- Repository URL:
https://github.com/yourusername/your-repo.git
- Script Path:
Jenkinsfile
3. Create the Jenkinsfile
In your GitHub repository, create a file named Jenkinsfile
with the following content:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
def app = docker.build("yourusername/yourapp:latest")
}
}
}
stage('Test') {
steps {
script {
app.inside {
sh 'npm test'
}
}
}
}
stage('Deploy') {
steps {
script {
kubernetesDeploy(configs: 'k8s/deployment.yaml', kubeConfig: [path: '/path/to/kubeconfig'])
}
}
}
}
}
This pipeline script performs three stages:
- Build: Builds the Docker image for the application.
- Test: Runs the application tests inside the Docker container.
- Deploy: Deploys the application to the Kubernetes cluster using a Kubernetes deployment configuration file.
Best Practices
1. Use Declarative Pipelines
Declarative pipelines are more readable and easier to maintain than scripted pipelines. Always prefer using declarative syntax for writing Jenkins pipelines.
2. Secure Sensitive Information
Use Jenkins credentials to manage sensitive information like API keys, passwords, and kubeconfig files. Avoid hardcoding credentials in your pipeline scripts.
3. Monitor Pipeline Performance
Regularly monitor and analyze pipeline performance to identify bottlenecks and optimize build and deploy times. Use Jenkins plugins like Performance Publisher and Monitoring Plugin for insights.
Lessons Learned from Real-World Implementations
Case Study: SaaS Product Deployment
A SaaS company implemented a Jenkins and Kubernetes-based CI/CD pipeline to streamline their deployment process. Initially, they faced issues with slow pipeline execution times. By optimizing the Docker build and leveraging parallel stages in Jenkins, they reduced build times by 50% and improved deployment reliability.
Pitfall: Lack of Rollback Strategy
One common pitfall is not having a rollback strategy in place. A company faced prolonged downtime due to deployment failures and the absence of a rollback mechanism. The lesson learned was to implement automatic rollback strategies using Kubernetes deployment configurations and Jenkins pipeline steps.
Conclusion
Using Jenkins with Kubernetes for CI/CD pipelines provides a robust and scalable solution for automating software delivery. By following best practices and learning from real-world experiences, you can optimize your CI/CD workflows and ensure reliable and efficient deployments. Have you implemented CI/CD pipelines with Jenkins and Kubernetes? Share your experiences and tips in the comments below!