Building a Serverless RESTful API with AWS Lambda and API Gateway
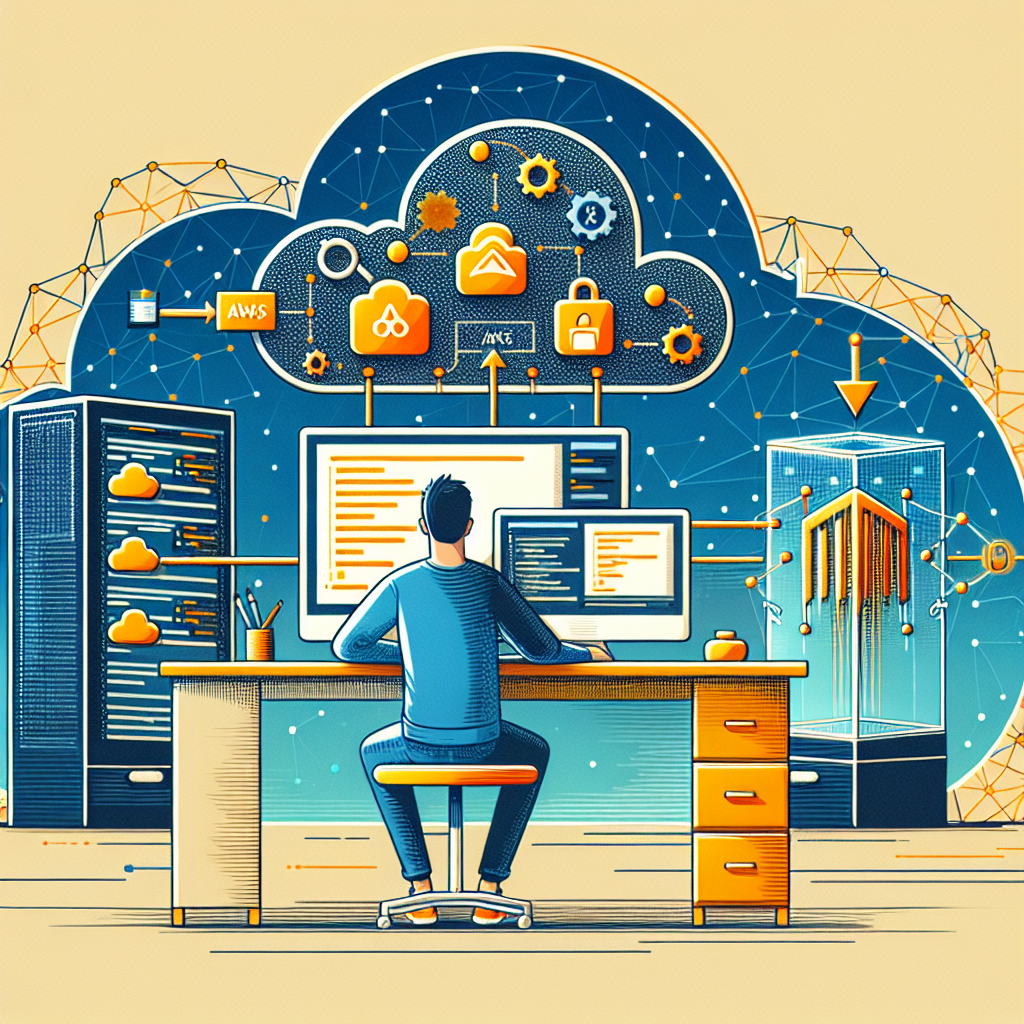
With the rise of cloud-native technologies, serverless computing has become a popular approach for building scalable applications without the need to manage infrastructure. AWS Lambda, a leading serverless computing service offered by Amazon Web Services, allows you to run code in response to events without provisioning or managing servers. In this blog post, we will explore how to build a serverless RESTful API using AWS Lambda and API Gateway, complete with code examples and step-by-step instructions.
Understanding Serverless Architecture
Serverless architecture allows you to build and run applications and services without thinking about servers. It eliminates the need for server management, helps you scale with demand, and lets you pay only for what you use. A serverless RESTful API is perfect for scenarios where you need a scalable solution to handle various HTTP requests without worrying about server maintenance.
Why AWS Lambda and API Gateway?
AWS Lambda and API Gateway are a powerful combination for creating serverless APIs. Here's why:
- Scalability: Automatically scales your API based on demand.
- Cost-Effective: You only pay for the compute time your functions use.
- Managed Service: No need to worry about server management.
- Seamless Integration: Easily integrates with other AWS services.
Building a Serverless RESTful API
Step 1: Set Up Your AWS Environment
Before we start, make sure you have an AWS account and that you have configured the AWS CLI on your local machine. You can follow the AWS CLI installation guide if you haven't done this yet.
Step 2: Create a Lambda Function
First, we need to create a Lambda function that will handle incoming HTTP requests. We will create a simple function in Python that returns a JSON response.
import json
def lambda_handler(event, context):
response = {
"statusCode": 200,
"body": json.dumps({
"message": "Hello, world!"
}),
}
return response
Save this code in a file named lambda_function.py
.
Step 3: Deploy the Lambda Function
Deploy the Lambda function using the AWS CLI:
aws lambda create-function --function-name HelloWorldFunction \
--runtime python3.8 \
--role arn:aws:iam::YOUR_ACCOUNT_ID:role/YOUR_ROLE_NAME \
--handler lambda_function.lambda_handler \
--zip-file fileb://function.zip
Ensure you replace YOUR_ACCOUNT_ID
and YOUR_ROLE_NAME
with your actual AWS account ID and IAM role name. You will need to create a zip file of your function:
zip function.zip lambda_function.py
Step 4: Set Up API Gateway
Now, we will create an API Gateway to expose our Lambda function as an API endpoint. You can do this through the AWS Management Console or using the AWS CLI. Here, we will use the AWS CLI for simplicity:
aws apigateway create-rest-api --name "HelloWorldAPI" --region us-east-1
Note the id
of the created API, as you will need it for subsequent commands.
Step 5: Create a Resource and Method
Create a resource and an HTTP method to handle incoming requests:
aws apigateway get-resources --rest-api-id YOUR_API_ID
aws apigateway create-resource --rest-api-id YOUR_API_ID \
--parent-id YOUR_PARENT_ID --path-part hello
aws apigateway put-method --rest-api-id YOUR_API_ID \
--resource-id YOUR_RESOURCE_ID --http-method GET \
--authorization-type "NONE"
Replace YOUR_API_ID
, YOUR_PARENT_ID
, and YOUR_RESOURCE_ID
with the appropriate values from your API Gateway setup.
Step 6: Integrate Lambda with API Gateway
Integrate the API Gateway resource with your Lambda function:
aws apigateway put-integration --rest-api-id YOUR_API_ID \
--resource-id YOUR_RESOURCE_ID --http-method GET \
--type AWS_PROXY \
--integration-http-method POST \
--uri arn:aws:apigateway:YOUR_REGION:lambda:path/2015-03-31/functions/arn:aws:lambda:YOUR_REGION:YOUR_ACCOUNT_ID:function:HelloWorldFunction/invocations
Remember to replace placeholders with your actual values.
Step 7: Deploy the API
Deploy your API to make it accessible over the internet:
aws apigateway create-deployment --rest-api-id YOUR_API_ID \
--stage-name prod
Step 8: Test Your API
Finally, test your API to ensure it works as expected:
curl -X GET https://YOUR_API_ID.execute-api.YOUR_REGION.amazonaws.com/prod/hello
You should get a JSON response: {"message":"Hello, world!"}
Lessons Learned
Building serverless APIs using AWS Lambda and API Gateway can significantly reduce the complexity and cost associated with managing infrastructure. However, it is crucial to handle various aspects such as IAM roles, API Gateway configurations, and AWS costs effectively. Here are some lessons learned:
- Proper IAM Permissions: Ensure that your Lambda functions have the appropriate IAM roles and permissions to interact with other AWS services securely.
- Monitoring and Logging: Enable CloudWatch logging for both Lambda and API Gateway to monitor and troubleshoot your serverless application effectively.
- Cost Management: Keep an eye on your AWS usage to avoid unexpected costs. Leverage AWS cost management tools to track and optimize your spending.
Conclusion
Serverless architecture, powered by AWS Lambda and API Gateway, provides a scalable and cost-effective way to build and deploy RESTful APIs. By following the steps outlined in this guide, you can quickly set up your serverless API and focus on building features rather than managing servers. Have you tried building serverless APIs with AWS Lambda and API Gateway? Share your experiences and tips in the comments below!